Generate React Native documentation: Using TypeScript and Typedoc
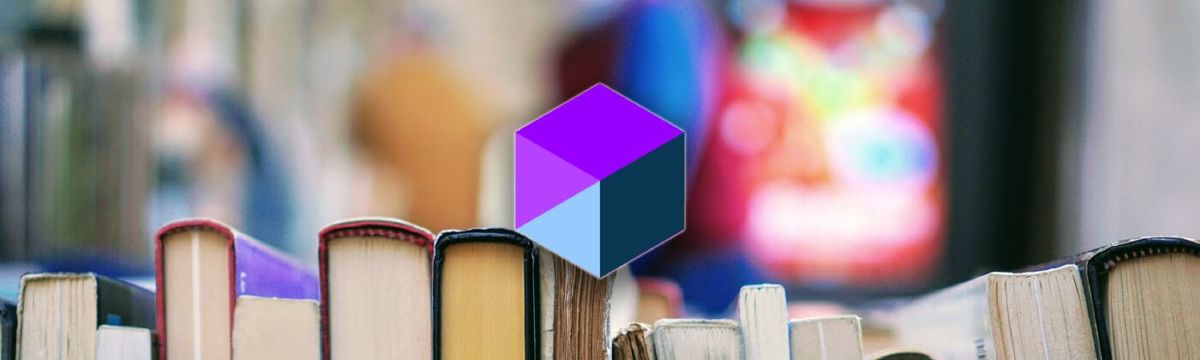
It is very common for a React Native project to end up incorporating hundreds of components, state slices and methods. A project can become very hard to maintain for any new or experienced developer if the architecture of the project isn't well documented, or just isn't there. On the other hand, writing and maintaining a documentation can mean days of work.
Luckily, it is possible to harness the power of TypeScript to automatically generate documentation in any React Native project. We will dive into the few steps needed to achieve this result.
TypeDoc runs on Node.js and is available as an npm package. You can save the package to your devDependencies:
$ npm install typedoc --save-dev
You can also install it as a global module and use it anywhere and in all your apps:
$ npm install typedoc --global
$ typedoc
From then on, you need to provide a configuration for the typedoc module to generate a documentation based on your needs. You can re-use your tsconfig.json
{
"compilerOptions": {
"target": "es2015",
"jsx": "react",
"noEmit": true,
"moduleResolution": "node",
"importHelpers": true,
"lib": ["es2015", "es2016"],
"allowSyntheticDefaultImports": true
},
"exclude": ["node_modules"]
}
Simply run this command to generate your documentation in the ./docs/documentation folder, looking for all files in ./src
$ typedoc --out ./docs/documentation ./src --mode file --tsconfig ./tsconfig.json
We almost instanly get our documentation generated as HTML files inside the specified folder.
There are many options to customize your generated documentation, which you could check the Typedoc Documentation for.
From then on you could add the command to your package.json scripts and have it ready for future uses:
"scripts": {
"start": "node node_modules/react-native/local-cli/cli.js start --config ../../../../rn-cli.config.js",
"android": "node node_modules/react-native/local-cli/cli.js run-android",
"test": "jest",
"documentation": "typedoc --out ./docs/documentation ./src --mode file --tsconfig ./tsconfig.json"
},
We can see how easy it is to automate the creation of a documentation when a project is set up using TypeScript. Apart from strong type-checking and solid boundaries, the JS superset proves once again its power for any Javascript project, from web to React Native.
If you want to read more about using TypeScript in React Native, check out this blog post about how to speed up React Native development using TypeScript: react-native-typescript-transformer.